Evaluate Reverse Polish Notation
Evaluate the value of an arithmetic expression in Reverse Polish Notation. Valid operators are +, -, *, /. Each operand may be an integer or another expression.
(计算逆波兰表达式)
Note:
- Division between two integers should truncate toward zero.
- The given RPN expression is always valid. That means the expression would always evaluate to a result and there won’t be any divide by zero operation.
Example:
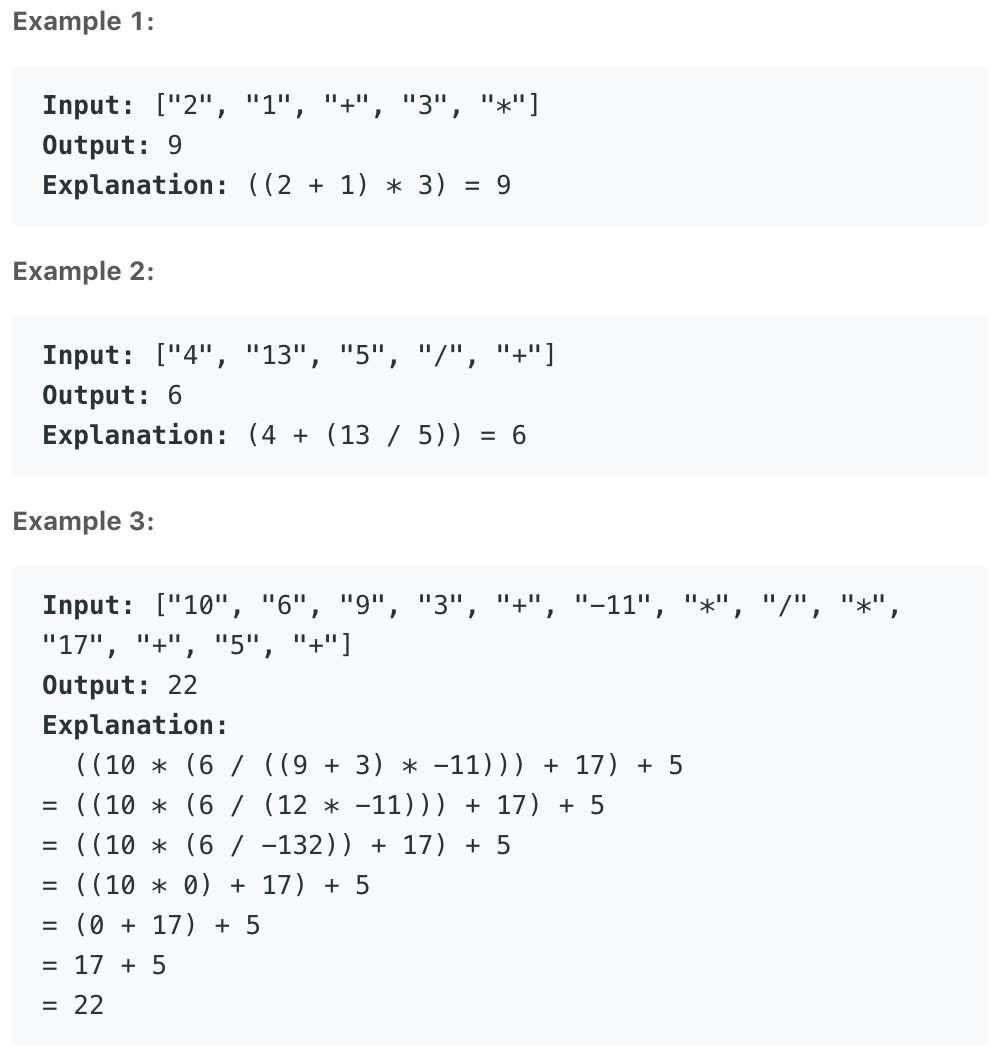
1. 栈
使用堆栈来计算逆波兰表达式。其中需要注意的是,题目中要求除法运算没有整除时要向 0 靠近,因此在两个异号的数相除时,应该 + 1 处理,或者转换为正数除法再加负号。具体实现过程如下:
1 | class (object): |